13 KiB
layout | title |
---|---|
slides | Slides: What's Next for Cap'n Proto |
What's Next for Cap'n Proto?
Cap'n Proto supports streaming!
{% highlight capnp %} interface FileStore { get @0 (name :Text, stream :Stream); put @1 (name :Text) -> (stream :Stream); }
interface Stream { write @0 (data :Data); end @1 (); } {% endhighlight %}
But flow control is up to the app.
Let's build it in.
{% highlight capnp %} interface Stream { write @0 (data :Data) -> bulk; end @1 (); } {% endhighlight %}
What about realtime streams?
{% highlight capnp %} interface VideoCallStream { sendFrame @0 (frame :Frame) -> realtime; } {% endhighlight %}
Best served on a UDP transport...
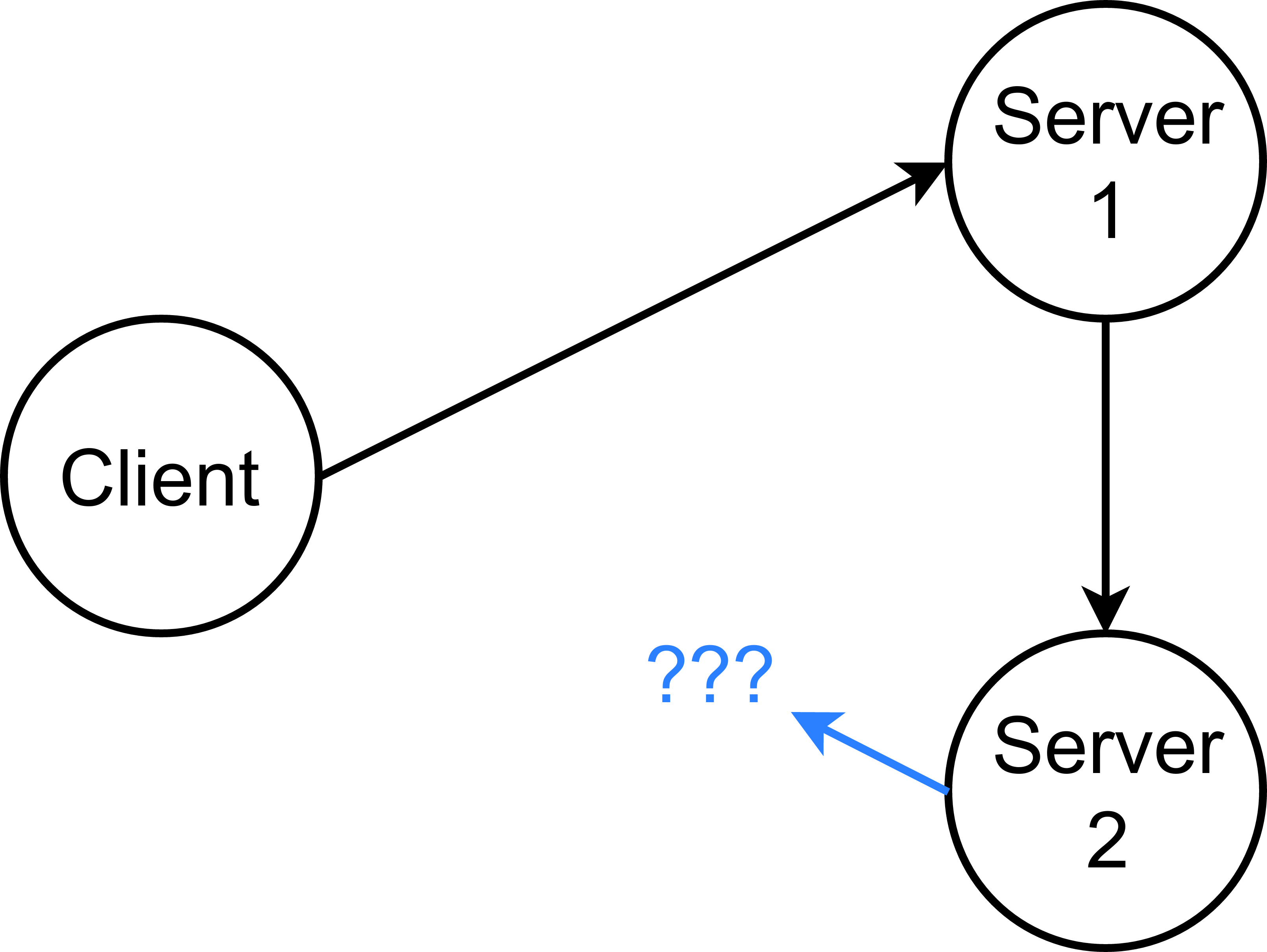
Forwarded request.
Where does response go?
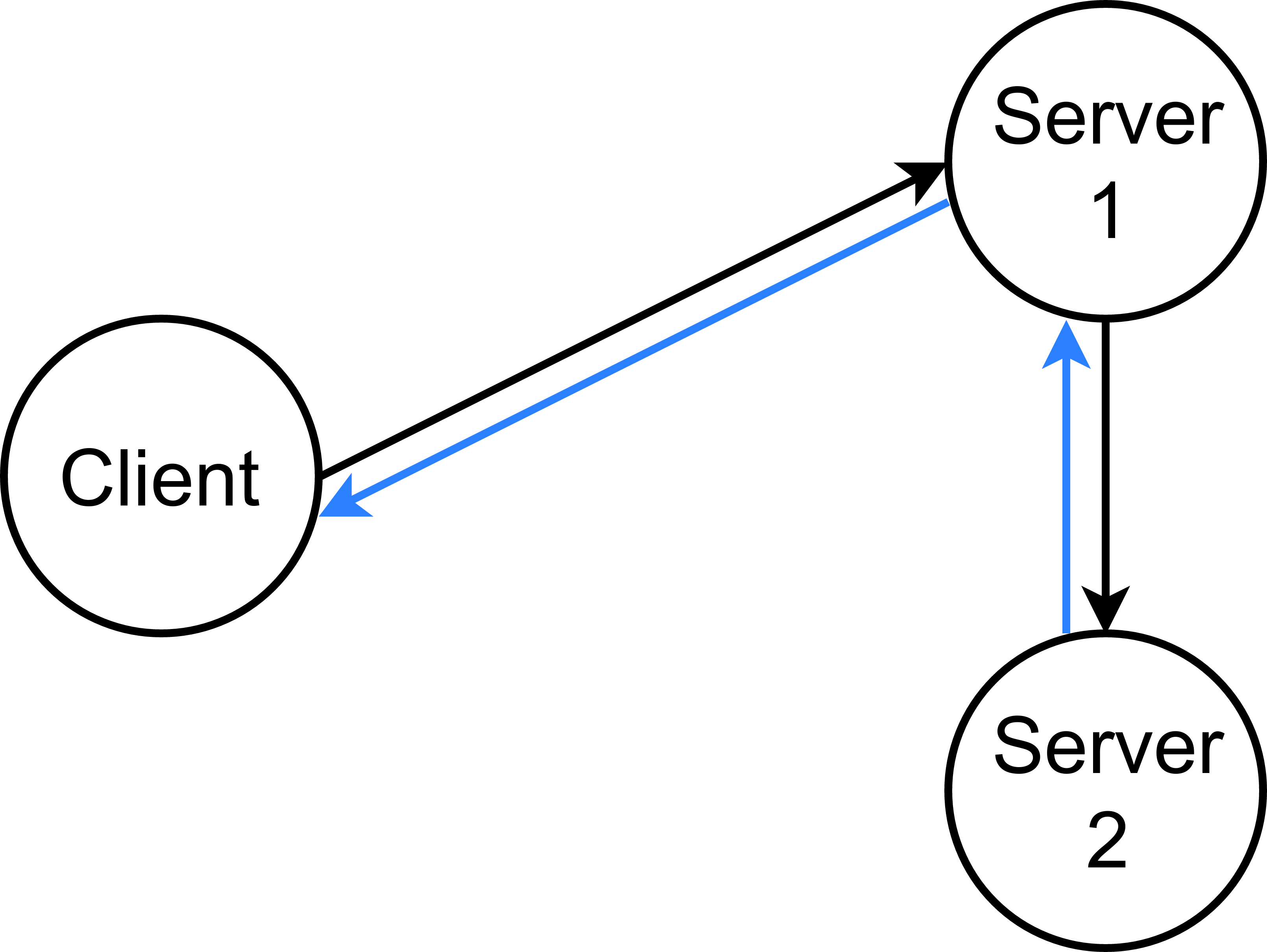
Classic solution:
Proxy

Classic solution:
Redirect
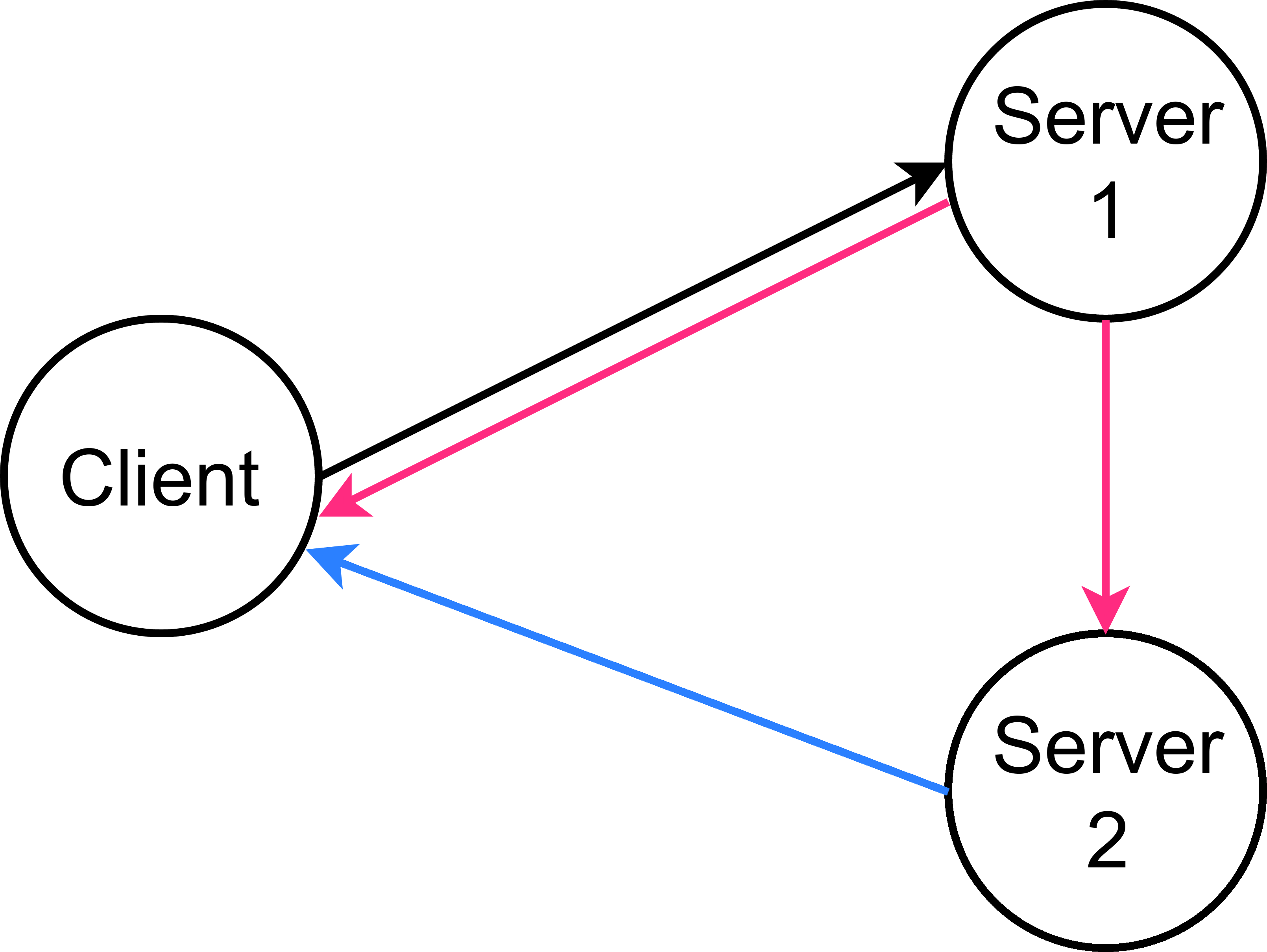
Cap'n Proto:
3-Party Handoff
(aka 3PH)
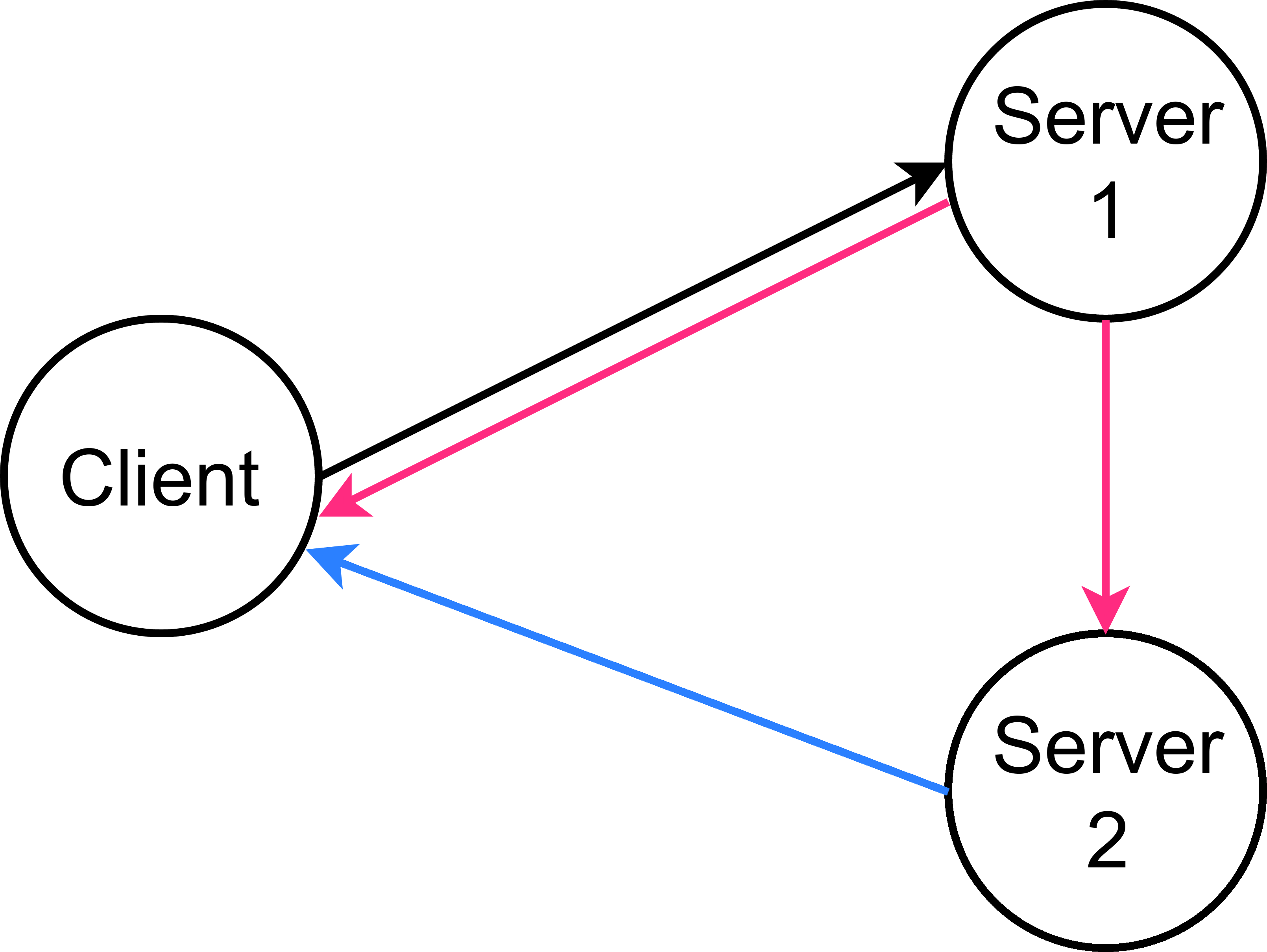
Cap'n Proto:
3-Party Handoff
(aka 3PH)
... gonna need UDP
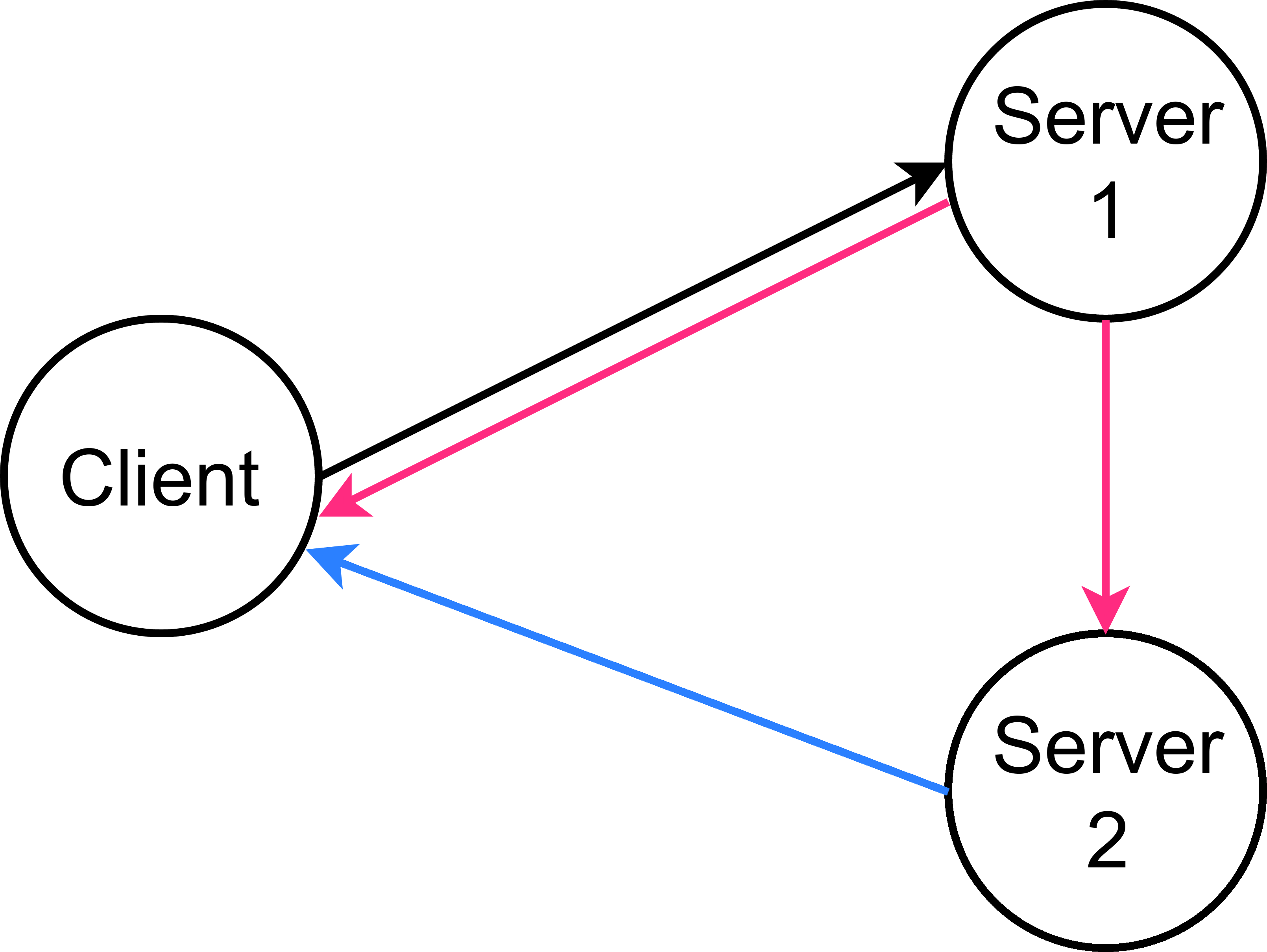
Cap'n Proto:
3-Party Handoff
(aka 3PH)
... gonna need UDP
... and 0-RT crypto
API: "Tail call"
{% highlight c++ %} kj::Promise myRpc(MyRpcContext context) override { // Begin sub-request. auto subRequest = someCapability.someRpcRequest(); subRequest.setSomeParam(someValue);
// Send as a tail call. return context.tailCall(kj::mv(subRequest)); } {% endhighlight %}
Today: Will proxy
Future: 3PH
KJ client networking, no TLS:
{% highlight c++ %} void send() { auto io = kj::setupAsyncIo(); auto& network = io.provider->getNetwork(); auto addr = network.parseAddress("capnproto.org", 80) .wait(io.waitScope); auto connection = addr->connect().wait(io.waitScope); connection->write("GET /", 5).wait(io.waitScope); } {% endhighlight %}
KJ client networking with TLS:
{% highlight c++ %} void send() { auto io = kj::setupAsyncIo(); kj::TlsContext tls; auto network = tls.wrapNetwork(io.provider->getNetwork()); auto addr = network->parseAddress("capnproto.org", 443) .wait(io.waitScope); auto connection = addr->connect().wait(io.waitScope); connection->write("GET /", 5).wait(io.waitScope); } {% endhighlight %}
Diff:
{% highlight c++ %} void send() {
kj::TlsContext tls; tls.wrapNetwork( );
} {% endhighlight %}
{% highlight c++ %} void receive() { auto io = kj::setupAsyncIo(); auto& network = io.provider->getNetwork(); auto addr = network.parseAddress("*", 80) .wait(io.waitScope); auto listener = addr->listen(); auto connection = listener->accept().wait(io.waitScope); connection->write("HTTP/1.1 404 Not Found\r\n\r\n", 26) .wait(io.waitScope); } {% endhighlight %}
{% highlight c++ %} void receive() { auto io = kj::setupAsyncIo(); kj::TlsKeypair keypair { KEY_PEM_TEXT, CERT_PEM_TEXT }; kj::TlsContext::Options options; options.defaultKeypair = keypair; kj::TlsContext tls(options); auto& network = io.provider->getNetwork(); auto addr = network.parseAddress("*", 443).wait(io.waitScope); auto listener = tls.wrapPort(addr->listen()); auto connection = listener->accept().wait(io.waitScope); connection->write("HTTP/1.1 404 Not Found\r\n\r\n", 26) .wait(io.waitScope); } {% endhighlight %}
{% highlight c++ %} void receive() {
kj::TlsKeypair keypair { KEY_PEM_TEXT, CERT_PEM_TEXT }; kj::TlsContext::Options options; options.defaultKeypair = keypair; kj::TlsContext tls(options);
tls.wrapPort( );
} {% endhighlight %}
{% highlight c++ %} auto io = kj::setupAsyncIo(); kj::HttpHeaderTable headerTable; auto client = kj::newHttpClient( *headerTable, io.provider->getNetwork());
kj::HttpHeaders headers(*headerTable); auto response = client->request( kj::HttpMethod::GET, "http://capnproto.org", headers) .response.wait(io.waitScope);
KJ_ASSERT(response.statusCode == 200); KJ_LOG(INFO, response.body->readAllText().wait(io.waitScope)); {% endhighlight %}
Headers identified by small numbers.
{% highlight c++ %} kj::HttpHeaderTable::Builder builder; kj::HttpHeaderId userAgent = builder.add("User-Agent"); auto headerTable = builder.build();
kj::HttpHeaders headers(*headerTable); headers.set(kj::HttpHeaderId::HOST, "capnproto.org"); headers.set(userAgent, "kj-http/0.6"); {% endhighlight %}
Header parsing is zero-copy.
Ugly imperative code:
{% highlight c++ %} capnp::MallocMessageBuilder message;
auto root = message.initRoot(); root.setFoo(123); root.setBar("foo"); auto inner = root.initBaz(); inner.setQux(true);
capnp::writeMessageToFd(fd, message); {% endhighlight %}
Nice declarative code:
{% highlight c++ %} using namespace capnp::init;
capnp::MallocMessageBuilder message; message.initRoot( $foo = 123, $bar = "foo", $baz( $qux = true ) ); capnp::writeMessageToFd(fd, message); {% endhighlight %}
Even better:
{% highlight c++ %} using namespace capnp::init;
capnp::writeMessageToFd(fd, $foo = 123, $bar = "foo", $baz( $qux = true ) ); {% endhighlight %}
{% highlight c++ %} struct { template struct Setter { T value; template void operator()(U& target) { target.setFoo(kj::fwd(value)); } };
template Setter operator=(T&& value) { return { kj::fwd(value) }; } } $foo; {% endhighlight %}
Not idiomatic:
{% highlight c++ %} capnp::MallocMessageBuilder message;
MyStruct::Builder root = message.initRoot(); root.setFoo(123); root.setBar("foo"); InnerStruct::Builder inner = root.initBaz(); inner.setQux(true);
capnp::writeMessageToFd(fd, message); {% endhighlight %}
Plain Old C++ Structs?
{% highlight c++ %} MyStruct root; root.foo = 123; root.bar = "foo"; InnerStruct inner; inner.qux = true; root.baz = kj::mv(inner);
capnp::writeMessageToFd(fd, message); {% endhighlight %}
Caveat: No longer zero-copy.
{% highlight c++ %} capnp::MallocMessageBuilder message; capnp::readMessageCopy(input, message); auto root = message.getRoot(); auto oldListOrphan = root.disownStructList(); auto oldList = oldListOrphan.getReader(); auto newList = root.initStructList(oldList.size() - 1); for (auto i: kj::indices(newList)) { newList.setWithCaveats(i, oldList[i < indexToRemove ? i : i + 1]); } capnp::MallocMessageBuilder message2; message2.setRoot(root.asReader()); capnp::writeMessage(output, message2); {% endhighlight %}
{% highlight c++ %} auto root = capnp::readMessageCopy(input); root.structList.erase(indexToRemove); capnp::writeMessageCopy(output, root); {% endhighlight %}
{% highlight capnp %} interface AddressBook { getPerson @0 (id :UInt32 $httpPath) -> (person :Person $httpBody(type = json)) $http(method = get, route = "person");
GET /person/
JSON response body
updatePerson @1 (id :UInt32 $httpPath, person :Person $httpBody(type = json)); $http(method = put, route = "person");
PUT /person/
JSON request body
} {% endhighlight %}
{% highlight capnp %} addPerson @2 (person :Person $httpBody(type = json)) -> (id :UInt32 $httpBody(type = jsonField)); $http(method = post, route = "person");
POST /person
JSON request body
JSON response body (object containing field id
)
getAll @3 (page :UInt32 = 0 $httpQuery) -> (people: List(Person) $httpBody(type = json)); $http(method = get);
GET /?page=
Query is optional.
JSAN (JSON array) repsonse body.
{% endhighlight %}
{% highlight capnp %} interface AddressBookService { getAddressBook @0 (key :String $httpPath) -> (result :AddressBook $httpPipeline); $http(route = "book");
GET /book/JrpmUduyHd8uW3x3TOXn2g/person/123
Becomes:
service.getAddressBook("JrpmUduyHd8uW3x3TOXn2g").send()
.getResult().getPerson(123).send()
GET /book/JrpmUduyHd8uW3x3TOXn2g
Becomes:
service.getAddressBook("JrpmUduyHd8uW3x3TOXn2g").send()
.getResult().getAll().send()
} {% endhighlight %}
Questions?